The error reporting built into PHP is crude, at best. Be it a parse error, the infamous “headers alr...
The error reporting built into PHP is crude, at best. Be it a parse error, the infamous “headers already sent,” or a “call to undefined function,” what you'll see is the error type and filename in bold, some technical jargon, and a line number that may or may not be correct.
But you don't have to rely upon PHP's error handling style. For a while now, PHP has allowed you to define your own error handler, such as:
```
<?php
function my_error_handler ($number, $message, $file, $line) {
// Match the formatting, CSS, etc., for your site's style!
echo 'The following error occurred at line ' . $line . ' of file ' . $file . ': ' . $message;
echo 'The existing variables are:' . print_r($GLOBALS, 1);
}
?>
```
Then you tell PHP to use your handler and not the default one by calling the set_error_handler() function.
```
<?php
set_error_handler('my_error_handler');
?>
```
From this point in your script forward, most errors will be handled by your function (there are some exceptions, including parse errors, which will still be handled the old way). With my example, the message is printed out with a little HTML formatting and, more importantly, all of the existing variables are printed within pre tags.
You might be thinking that I didn't really do anything novel with print_r() in my error handler. True, but I could just as easily build up a detailed error message that is then e-mailed to me should a problem occur on a live site (when you shouldn't display this information to the site's user).
Just in case that technique is a bit of a yawner to you, I'll also mention this: Along with the many new features in PHP 5, another method of error handling has been introduced, in keeping with C++/Java/C# style. This format uses the try-catch syntax:
```
<?php
try {
if (!@mysql_connect('localhost', 'username', 'password'))
throw new Exception (mysql_error());
} catch (Exception $e) {
echo 'Could not connect to the database because: ' . $e->getMessage();
}
?>
```
Of course that's just a basic example; there's a lot more than you can do with this method of error handling. In particular, if you're comfortable with object oriented programming (OOP), you can define and use your own Exception class. Or you can have multiple catch statements, each catching a different type of exception.
If you are interested in reading our other blog posts, check them out on our website. If you have any questions, feel free to contact our support team and we will be more than happy to assist you.
Don't forget to follow us on Twitter for news, updates and announcements - @vexxhost.
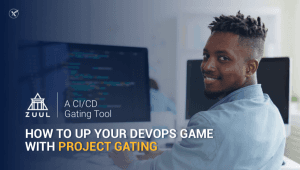